Git Commands
📚 Table of Contents |
---|
Create a repo |
Clone a repo |
Making changes |
Pull changes |
Branches |
Undo changs |
- to understand the git commands, you need to have a grasp on the basic git workflow
create or clone a repo > stage changed files > commit staged files > push changed files > pull changed files
Create a git repo
- first you need a repository (code base) to work with. you have two options off the bat, you can create a git repo with your own code or you can clone an existing repo
- if you are using the GitHub Desktop app, you can "Create a New Repository", which will create a new project on your computer with git or you can add git to a project you already have with "Add Existing Repository"
- the GitHub Desktop app will automatically publish (create the repo) on GitHub if you are creating or adding a repo. otherwise, you will have to go to github.com (opens in a new tab) and create the repo yourself
- if you are doing this sans the desktop app, you will have to initialize git in a local (on your computer) project folder/directory and then push the project to GitHub yourself
- in the project directory that you are adding:
$ git init
- now you need to push the code to GitHub
$ git remote add origin [url]
- the url would be where you made the project on GitHub; something like https://github.com/yourusername/yourepository (opens in a new tab)
Clone a repo
- you clone a repo when you are invited to work on a repo that is not under your account. that could mean your company's (or friend's) project or if you are going to contribute to an open source project
- the process is very easy on GitHub if you have the CLI setup (like we did on the previoue page). click that green "Code" button and copy the CLI command and paste it into your terminal:
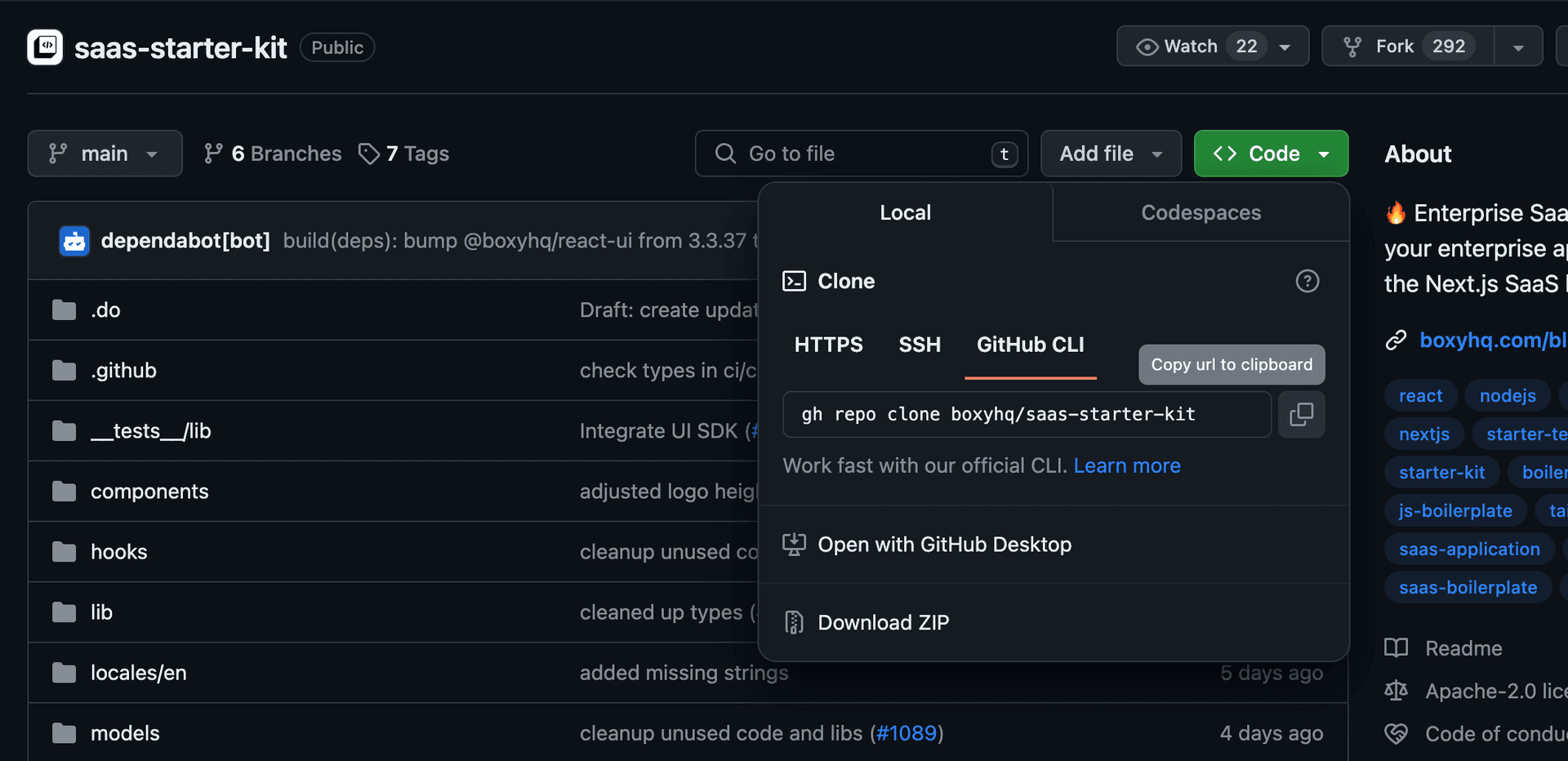
$ gh clone repo [username/respositoryname]
- that will create a directory with all of the files on your computer and will be tied to that specific repo
- again, cloning a repo means you are now connected to that specific repo under that other account and the changes you make will get pushed to that repo. if you want to just grab the code and make your own repo you can do that a few ways too
- if you want to bring that code into a repo under your own account, you can fork a copy of it by clicking the "Fork" button right above the green "Code" button
- or you cloned into the repo and don't want to be tied to the original remote origin, you can either change it and point it to your own repo:
$ git remote set-url origin https://github.com/yourusername/yourrepository.git
- or remove the link to the remote origin completely:
$ git remote remove origin
Make some changes
- cool, so now you would have your project setup and connected to a repo. if you make any changes from adding new files to changing a single character on a line of code you can check that git is tracking them with:
$ git status
- that will show you the files you have modified. they probably will be in red to let you know those files are not staged yet
- you can stage the changed individual files one at a time:
$ git add [file]
- or you can stage all changed files at the same time:
$ git add .
- make sure they are staged with
git status
again and they should be green now - or if you're using the desktop app, you'll notice its already tracking and staged your changed files 😎
- next, on either the desktop app or in the terminal, you'll need to commit your changed files and add a mandatory comment
- the comments aren't supposed to be long, something like "fixed the submit button bug" or "changed heading to blue" or "updated dependencies", you get it. fun fact some apps like Jira use these comments to show your tech leads and product managers exactly what you have been working on, so its a good idea not to get into the habit of making comments like "fixed some shit"
- make your comment in the desktop app and click "commit" or do it in the terminal:
$ git commit -m "put your comment here"
- and finally the most satisfying step in this little process. push those changes and upload them to GitHub. in the desktop app, click the "Push origin" button or in the terminal:
$ git push
Pull changes
- if somebody else made changes to the repo, it's a good idea to pull their changes into your code. again, theres a few ways to do it
- the manual more incremental way would be to fetch the changes your partner made, review them and then merge them into your code. this is definitely helpful if you are worried they changed something you could be working on
$ git fetch
- then if everything looks cool to you, merge it into your code:
$ git merge origin main
-
main
is the branch (it used to be called "master" but now it will always bemain
), so if you are working on a different branch, make sure you're fetching and merging the right one -
if you're feeling confident that your partners work won't mess yours up, you can fetch and merge their code into yours with one command:
$ git pull
- you may have heard of Pull Requests or PR's. that is when you make changes and are requesting approval for your code to be merged in by the owner. you do that on the platform through the website
Branches
- branches are a huge part of working with git
- like I mentioned in the intro part, they let you work on code or create new code without affecting the main project until you it is ready
- switching between branches, creating new ones and merging branches is pretty self explanatory in the desktop app but heres how you do it in the terminal:
- check what branch you are currently on:
$ git branch
- create a new branch:
$ git branch [branch-name]
- switch branches and update the directory:
$ git checkout [branch-name]
- to merge branches, run the following command but know that you are merging into the one you are currently on, so its a good idea to check
git status
and/orgit branch
first:
$ git merge [branch-name]
Undo changes
- you committed code at 5:00pm on a friday to the main branch and just took down production. you aren't sure wtf you did and can't fix it quickly. you need to undo your commit fast:
$ git reset [commit]
- that will undo all commits made after the one you specified and preserve your local code. if you need to all history and changes back run this:
$ git reset --hard [commit]
- ⚠️ note: a message straight from GitHub - "CAUTION! Changing history can have nasty side effects. If you need to change commits that exist on GitHub (the remote), proceed with caution. If you need help, reach out at github.community (opens in a new tab) or contact support." so, try to save this for emergency situations but know that it is possible